A servicedesk employee came to me with a problem with the autocomplete functionality in Outlook 2010. So I looked around for a bit to find some code. I came across a script that does the job. I added functionality so that it would work for the servicedesk. For the script to work, the user will need to have enough permissions to add permissions to any mailbox. The script is semi-automatic. So user interaction is needed.
Outlook 2003 used to have NK2 files which are corrupt from time to time. Then Microsoft replaced that functionality into a .dat with exact the same issues. Clearly in Outlook 2010 this problem still exists. The key difference is that Outlook 2010 and Outlook 2013 in combination with Exchange 2010 or later has a functionality called suggested contacts. This works similar to the autocomplete function only better. Contacts added to the suggested contacts will be in the autocomplete list. Imagine that by generating a list of all unique recipients in the sent item you’ll have a list that is satisfactory for the user.
Furthermore this functionality works regardless of the user profile. So in our case if a user uses Outlook Web App that user will also have that same functionality. There is a catch. This does not work as intended in combination with Res Workspace Manager and Zero-profiling. To get this right you can use another script.
This script gives write the LegacyDN as output on screen. This is needed for situaties where you have GAL segregation. Then it opens the control panel for e-mail on that server. Of course Outlook needs to be installed on the system. That you need to permit the script to use Outlook. You’ll get the warning as seen below:
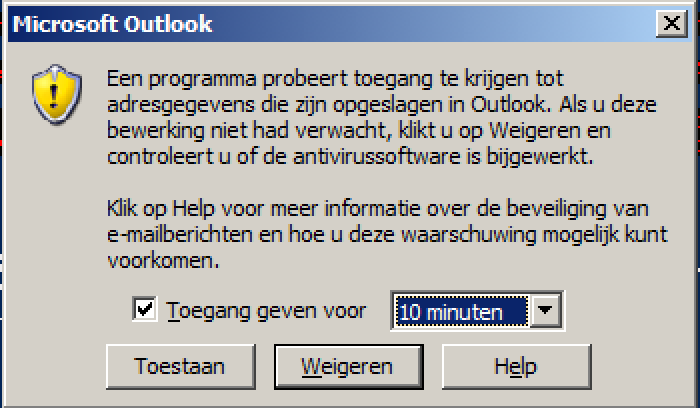
Again if you use RES Workspace Manager with Zero-Profiling use another script.
#Import Modules,Snapins and functions
ipmo ActiveDirectory
Add-PSSnapin Microsoft.Exchange.Management.Powershell.E2010
Function AddTo-SuggestedContactsIfNotAlreadyAdded ($name, $email)
{
if(($name -eq "") -or ($email -eq "") -or ($name -eq $null) -or ($email -eq $null)){
return;
}
$name = $name.Replace("'", "").Replace("""", "")
$contactAlreadyAdded = $false
foreach ($elem in $global:alreadyAddedEmails) {
if(($elem.ToLower() -match $email.ToLower())){
$contactAlreadyAdded = $true
Write-Host ($global:counter)"/"($totalItems) "SKIPPED " $name.PadRight(25," ") "-" $email
return;
}
}
if(!$contactAlreadyAdded ) {
$newcontact = $contacts.Items.Add()
$newcontact.FullName = $name
$newcontact.Email1Address = $email
$newcontact.Save()
$global:alreadyAddedEmails += $email
Write-Host ($global:counter)"/"($totalItems) "ADDED " $name.PadRight(25," ") "-" $email
}
}
#Set Variables
$Admin = [Environment]::UserName
$choice2 = "y"
$Admindomain = $env:userdomain
#Set loop function
while ($choice2 -match "y"){
#Request Username from interactive User
Write-Host "Voer de gebruikersnaam van de gebruiker in: " -NoNewline -F Cyan
$Username = read-host
#Test if user exist
$Testresult = $Null
$Testresult = Get-ADUser $Username -Properties *
While ($Testresult -eq $Null){
write-host "De opgegeven Username bestaat niet of is foutief ingevoerd. Toets de username in in het format zoals bijvoorbeeld OTHSBE02" -b Black -f Red
$Username = Read-Host "Voer de gebruikersnaam van de gebruiker in:" -NoNewline -F Cyan
$Testresult = Get-ADUser $Username -Properties *}
Get-ADUser -Identity $Testresult.samaccountname -Properties legacyExchangeDN | Select legacyExchangeDN
#Addmailbox permission to the admin user with retry
Add-MailboxPermission -Identity $Testresult.samaccountname -AccessRights "FullAccess" -User ("$AdminDomain"+"\"+"$Admin")
$Testresult2 = $null
$Testresult2 = Get-MailboxPermission -Identity $Testresult.samaccountname | ? {$_.User -match ("$AdminDomain"+"\\"+"$Admin")}
While ($Testresult2 -eq $Null){
write-host "De rechten op de mailbox zijn niet goed gezet. Het wordt nogmaals geprobeerd" -b Black -f Red
Add-MailboxPermission -Identity $Testresult.samaccountname -AccessRights "FullAccess" -User ("$AdminDomain"+"\"+"$Admin")
$Testresult2 = Get-MailboxPermission -Identity $Testresult.samaccountname | ? {$_.User -match ("$AdminDomain"+"\\"+"$Admin")}}
#Opens Mail control panel
C:\Windows\SysWOW64\control.exe mlcfg32.cpl
#Confirm that the admin have changed to Exchange profile accordingly.
$choice = $Null
while ($choice -notmatch "[y|n]"){
$choice = read-host "Heb je het Outlook profiel aangepast? (Y/N)"
}
$outlook = new-object -com outlook.application
$olFolders = "Microsoft.Office.Interop.Outlook.OlDefaultFolders" -as [type]
$namespace = $outlook.GetNameSpace("MAPI")
$sentItems = $namespace.getDefaultFolder($olFolders::olFolderSentMail)
$alreadyAddedEmails = @() #Empty Array
$counter = 0;
$totalItems = $sentItems.items.count;
Write-Host "Scanning through" $totalItems "emails in SentItems"
$contacts = $outlook.Session.GetDefaultFolder($olFolders::olFolderSuggestedContacts)
# Loop through all emails in SentItems
$sentItems.Items | % {
#Loop through each recipient
$_.Recipients | %{
AddTo-SuggestedContactsIfNotAlreadyAdded $_.Name $_.Address
}
$global:counter = $global:counter + 1
}
$outlook.Quit()
#Addmailbox permission to the admin user with retry
Remove-MailboxPermission -Identity $Testresult.samaccountname -AccessRights "FullAccess" -User ("$AdminDomain"+"\"+"$Admin")
$Testresult2 = Get-MailboxPermission -Identity $Testresult.samaccountname | ? {$_.User -match ("$AdminDomain"+"\\"+"$Admin")}
While ($Testresult2 -ne $Null){
write-host "De rechten op de mailbox zijn niet goed gezet. Het wordt nogmaals geprobeerd" -b Black -f Red
Remove-MailboxPermission -Identity $Testresult.samaccountname -AccessRights "FullAccess" -User ("$AdminDomain"+"\"+"$Admin")
$Testresult2 = Get-MailboxPermission -Identity $Testresult.samaccountname | ? {$_.User -match ("$AdminDomain"+"\\"+"$Admin")}}
#Present user with the choice to do another user.
$choice2 = read-host "Wilt u dit voor nog een user uitvoeren? (Y/N)"
}